From New Scientist #1933, 9th July 1994 [link]
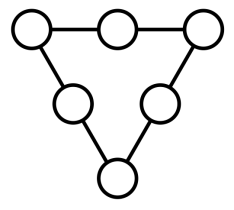
Put a digit into each circle and read each side of the triangle clockwise, as a three-figure number. (For example, if I put a 2 in the top left-hand corner and continued, clockwise, 4, 6, 1, 4, 9, then the three-figure numbers would be 246, 614 and 492: notice that 492 is a multiple of 246, but 614 isn’t).
Your job is to choose the digits so that the second and third three-figure numbers are different multiples of the first three figure number.
What are the numbers in your triangle (clockwise from the top left-hand corner)?
[enigma778]
It is straightforward to use the [[
SubstitutedExpression
]] solver from the enigma.py library to solve this puzzle.The following run file executes in 81ms. (Internal runtime of the generated program is 8.2ms).
Run: [ @replit ]
Solution: The triangle looks like this:
And we have:
This is my code:
Here’s an extremely performant solution (less then 1 msec on my computer):
Very fast, one minor error (e.g. for side1 125 the calculated side2 is too high). I adapted your version to this:
Thanks for the bug fix and improvements.
Three times faster according to multiple runs with timeit.